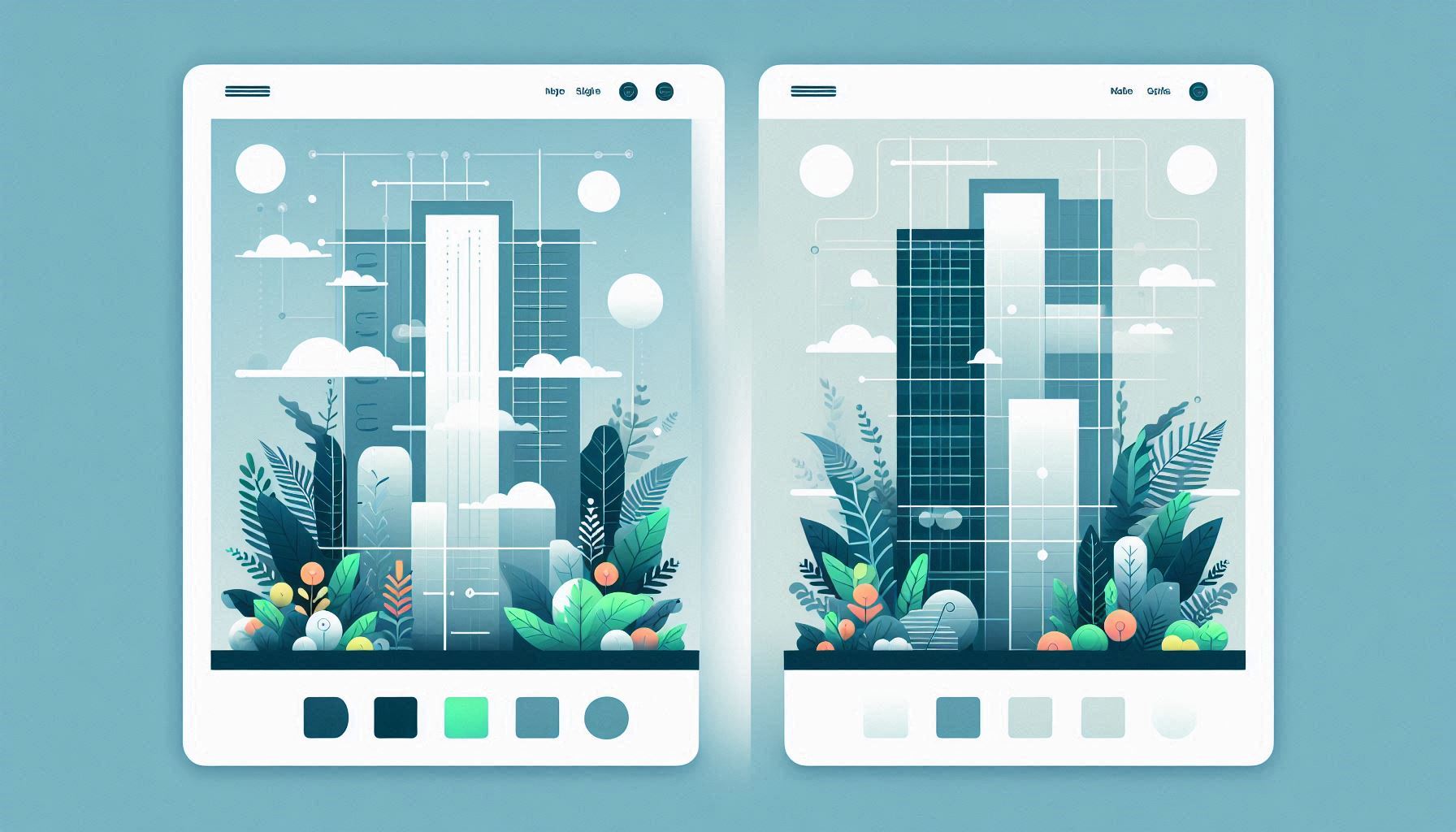
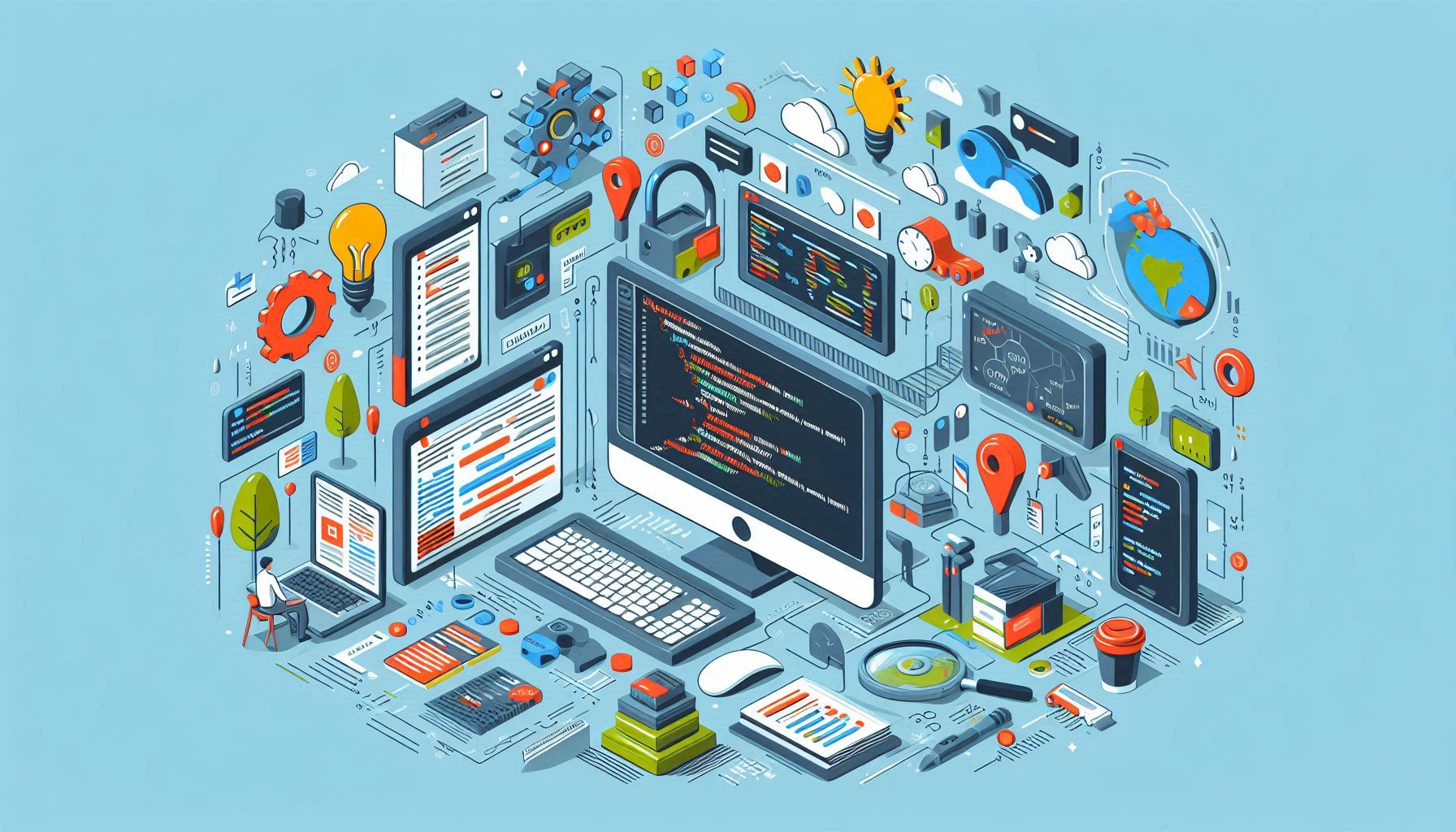
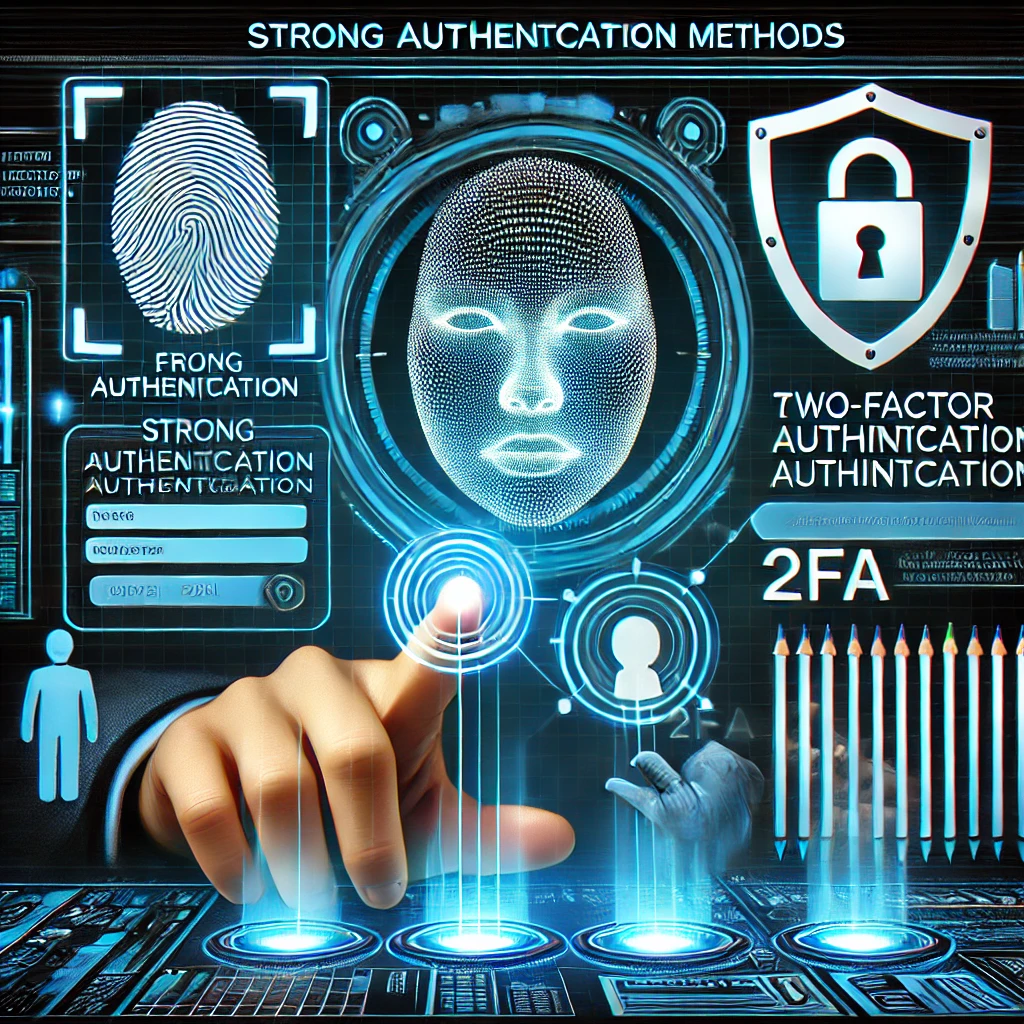
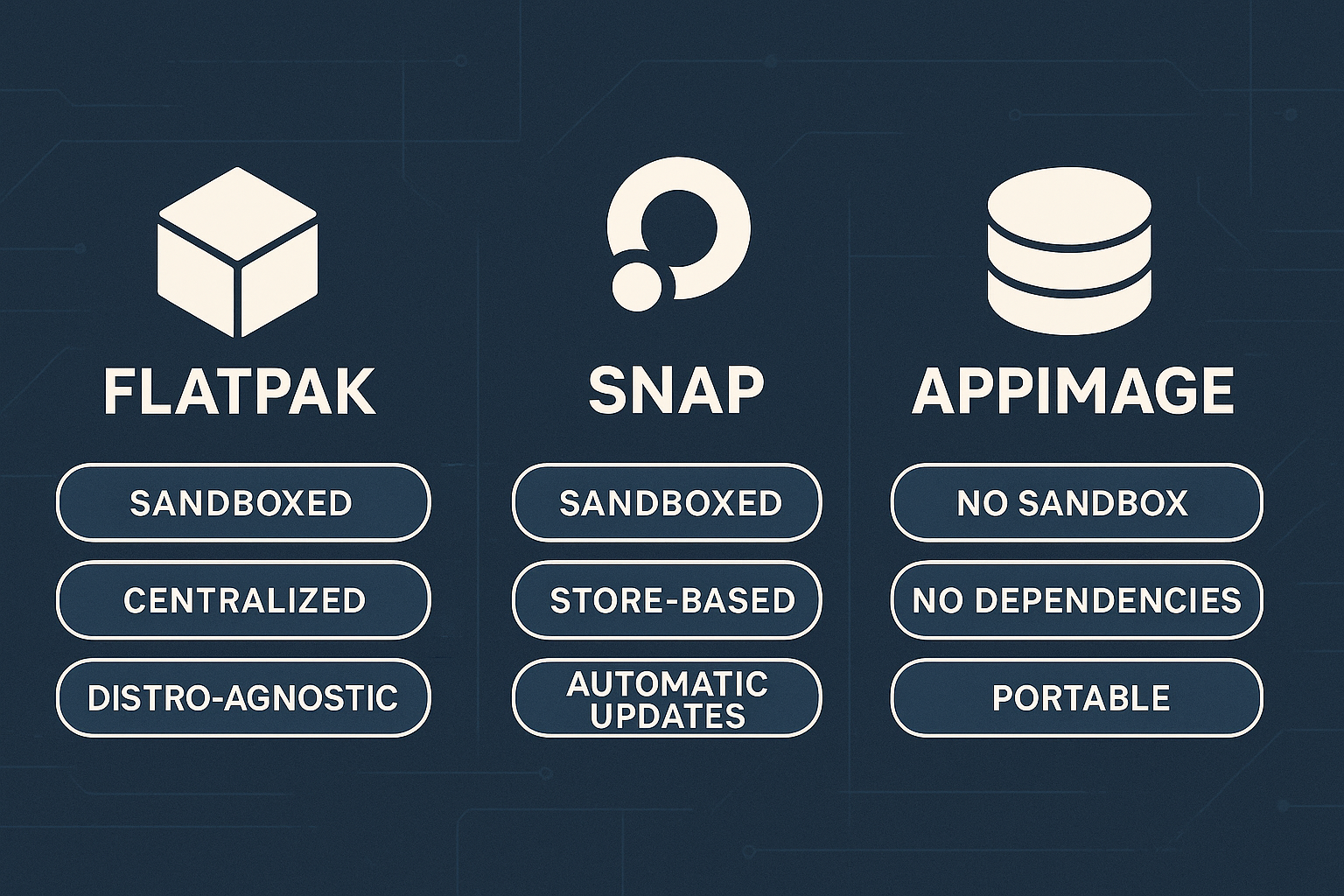
PHP, binlerce yerleşik (built-in) fonksiyonla gelir. Bunlar, verileri işlemekten filtrelemeye, dizilerle çalışmaktan metin düzenlemeye kadar birçok iş...
Modern web tasarımında düzen (layout) oluştururken iki güçlü CSS aracı öne çıkar: Flexbox ve Grid. Her ikisi de öğeleri hizalamak ve düzenlemek için k...
Mobil uyumlu web siteleri artık bir seçenek değil, zorunluluk. Farklı ekran boyutlarında doğru görüntü sunmak için responsive (duyarlı) tasarım teknik...
Hepimiz interneti sıkça kullanıyoruz ve neredeyse her gün bir yere giriş yapmak için şifre kullanıyoruz. Ama günümüzde sadece şifreler yeterli de...
Siber saldırılar, günümüzde bireylerden büyük şirketlere kadar herkes için ciddi bir tehdit oluşturmaktadır. Bir sistemin saldırıya uğraması, ver...
Firebase İncelemesi: Modern Uygulamalar İçin Gerçek Zamanlı Backend Platformu Google tarafından geliştirilen Firebase, özellikle mobil ve w...
Canva İncelemesi: Tasarım Bilgisi Olmadan Harika Görseller Oluşturun Tasarım yapmayı bilmeden profesyonel görseller, sunumlar, sosyal medya...
Web sitelerini barındırmak, alan adlarını yönetmek ve sunucu kaynaklarını kontrol etmek artık oldukça kolay. Nasıl mı? Cevap: cPanel & WHM! Özelli...