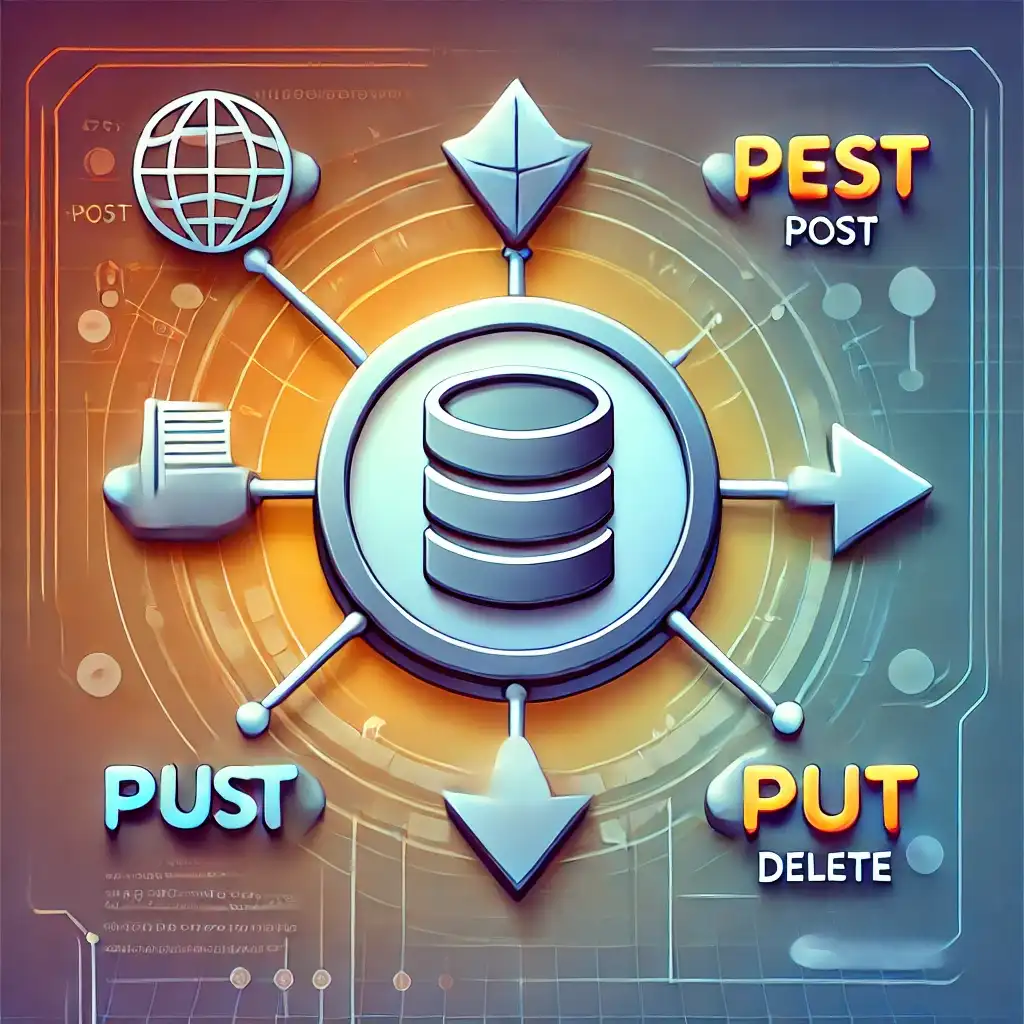
RESTful API, web servisleri oluşturmak için yaygın olarak kullanılan bir yaklaşımdır. PHP kullanarak RESTful API geliştirmenin temel adımlarını anlatmaya çalıştım ve örneklerle destekledim. Umarım anlarsınız..
Yeni Kullanıcı Ekleme:
1. RESTful API Temelleri
REST (Representational State Transfer), HTTP protokolü üzerinden veri alışverişi yapmak için kullanılan bir mimaridir. İşlevleri genellikle HTTP metodları ile belirlenir:- GET - Veri okuma
- POST - Yeni veri ekleme
- PUT - Mevcut veriyi güncelleme
- DELETE - Veri silme
2. PHP ile RESTful API Geliştirme
2.1. API Dizini Yapısı
API dosyalarını düzenli tutmak için aşağıdaki dizin yapısını kullanabiliriz:
GENEL
/api
|-- index.php
|-- config.php
|-- database.php
|-- routes.php
|-- controllers
|-- UserController.php
|-- models
|-- User.php
2.2. Veritabanı Yapılandırması (MySQL Kullanımı)
Öncelikle, aşağıdaki SQL sorgusunu kullanarak veritabanı veusers
tablosunu oluşturalım:
SQL
CREATE DATABASE testdb;
USE testdb;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL UNIQUE,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
2.3. Veritabanı Bağlantısı (database.php)
PHP
class Database {
private $host = 'localhost';
private $db_name = 'testdb';
private $username = 'root';
private $password = '';
public $conn;
public function getConnection() {
$this->conn = null;
try {
$this->conn = new PDO('mysql:host=' . $this->host . ';dbname=' . $this->db_name, $this->username, $this->password);
$this->conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $exception) {
echo 'Connection error: ' . $exception->getMessage();
}
return $this->conn;
}
}
2.4. Kullanıcı Modeli (models/User.php)
PHP
class User {
private $conn;
private $table_name = 'users';
public $id;
public $name;
public $email;
public $password;
public function __construct($db) {
$this->conn = $db;
}
public function read() {
$query = 'SELECT id, name, email, created_at FROM ' . $this->table_name;
$stmt = $this->conn->prepare($query);
$stmt->execute();
return $stmt;
}
public function create() {
$query = 'INSERT INTO ' . $this->table_name . ' (name, email, password) VALUES (:name, :email, :password)';
$stmt = $this->conn->prepare($query);
$stmt->bindParam(':name', $this->name);
$stmt->bindParam(':email', $this->email);
$stmt->bindParam(':password', $this->password);
if ($stmt->execute()) {
return true;
}
return false;
}
}
2.5. Kullanıcı Kontrolcüsü (controllers/UserController.php)
PHP
include_once '../database.php';
include_once '../models/User.php';
$database = new Database();
$db = $database->getConnection();
$user = new User($db);
if ($_SERVER['REQUEST_METHOD'] === 'GET') {
$stmt = $user->read();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
echo json_encode($users);
} elseif ($_SERVER['REQUEST_METHOD'] === 'POST') {
$data = json_decode(file_get_contents('php://input'));
$user->name = $data->name;
$user->email = $data->email;
$user->password = password_hash($data->password, PASSWORD_DEFAULT);
if ($user->create()) {
echo json_encode(['message' => 'User created successfully']);
} else {
echo json_encode(['message' => 'Unable to create user']);
}
}
2.6. API Ana Dosya (index.php)
PHP
header('Access-Control-Allow-Origin: *');
header('Content-Type: application/json; charset=UTF-8');
header('Access-Control-Allow-Methods: GET, POST');
header('Access-Control-Allow-Headers: Content-Type');
include_once 'controllers/UserController.php';
3. API Test Etme
API'nizi test etmek için Postman veya cURL kullanabilirsiniz. Kullanıcıları Listeleme:
SH
curl -X GET http://localhost/api/index.php
SH
curl -X POST http://localhost/api/index.php \
-H 'Content-Type: application/json' \
-d '{'name': 'John Doe', 'email': 'john@example.com', 'password': '123456'}'
4. API Güvenliği
- Güvenli Bağlantı Kullanın: API'yi HTTPS ile sunun.
- Kimlik Doğrulama (JWT Kullanımı): Kullanıcı kimlik doğrulama için JWT entegrasyonu yapın.
- Rate Limiting: Saldırılara karşı istek limitlemesi ekleyin.
- Girdi Doğrulama: SQL Injection ve XSS gibi siber tehditlere karşı verileri filtreleyin.
Benzer Yazılar
Yorumlar ()
Henüz yorum yok. İlk yorum yapan sen ol!