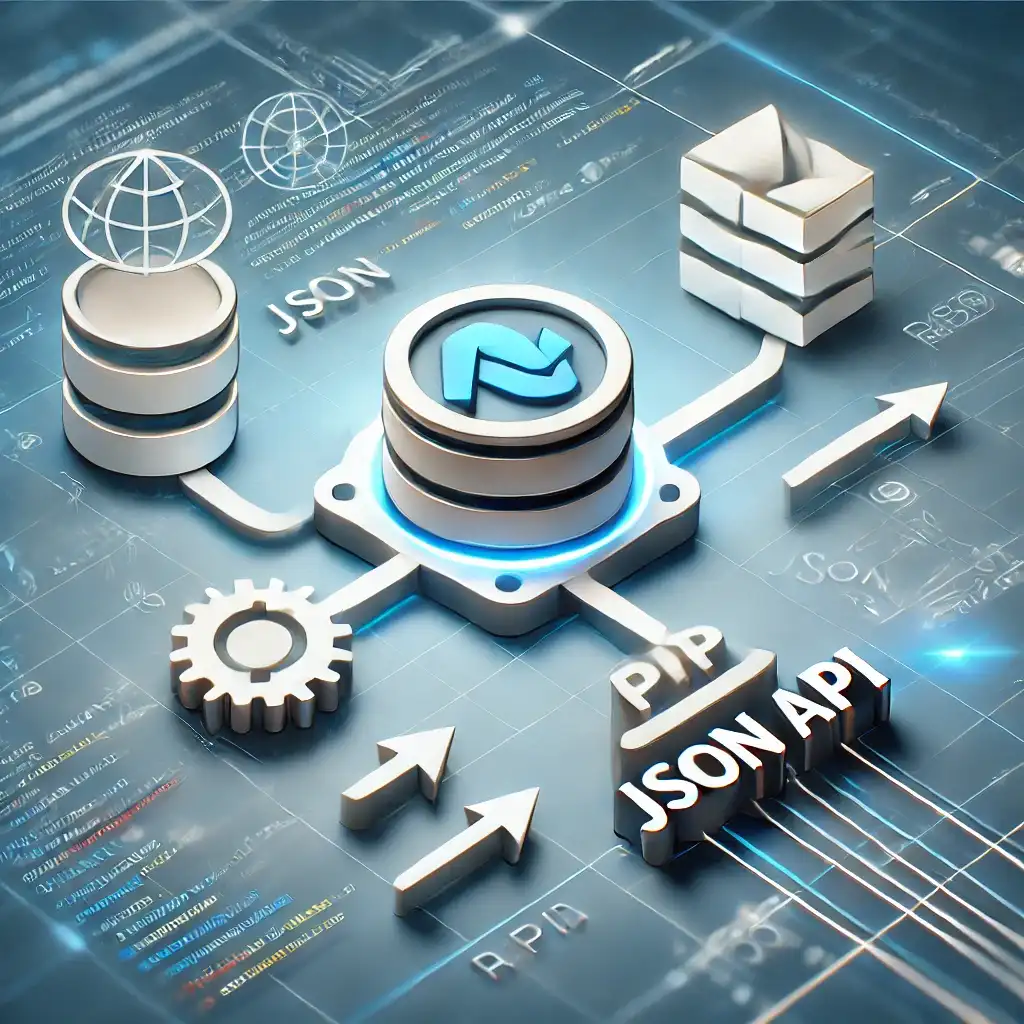
Web geliştirmede API’ler, farklı sistemlerin veri alışverişini sağlayan en önemli araçlardan biridir. JSON (JavaScript Object Notation), API’ler tarafından veri gönderme ve alma işlemleri için kullanılan en yaygın formattır. PHP ile harici bir API’den JSON veri çekebilir, bu veriyi işleyerek web projelerinizde kullanabilirsiniz.
Bu yazıda, PHP ile JSON API kullanımı, veri çekme ve işleme yöntemlerini anlatacağım.
PHP ile JSON API kullanımı, veri alışverişini yönetmek ve dinamik uygulamalar oluşturmak için kritik bir beceridir. Bu bilgilerle PHP ve JSON API entegrasyonu konusunda daha güçlü uygulamalar geliştirebilirsiniz!
1. PHP’de JSON Veri Formatı Kullanımı
PHP, JSON verileri oluşturmak ve işlemek için json_encode() ve json_decode() gibi yerleşik fonksiyonlar sunar.JSON Encode (PHP Dizisini JSON’a Dönüştürme)
PHP
$data = array(
'isim' => 'Ahmet',
'yas' => 30,
'meslek' => 'Yazılım Geliştirici'
);
$jsonVeri = json_encode($data, JSON_PRETTY_PRINT);
echo $jsonVeri;
- ✅ Çıktı:
JSON
{
'isim': 'Ahmet',
'yas': 30,
'meslek': 'Yazılım Geliştirici'
}
JSON Decode (JSON Verisini PHP Dizisine Çevirme)
PHP
$jsonVeri = '{'isim':'Ahmet','yas':30,'meslek':'Yazılım Geliştirici'}';
$phpDizisi = json_decode($jsonVeri, true);
print_r($phpDizisi);
- ✅ Çıktı:
PHP
Array (
[isim] => Ahmet
[yas] => 30
[meslek] => Yazılım Geliştirici
)
2. PHP ile Harici API’den JSON Veri Çekme
PHP’de API’den veri çekmek için cURL (Client URL Request Library) veya file_get_contents() gibi yöntemler kullanılabilir.Basit Bir API’den JSON Veri Çekme (file_get_contents)
PHP
$url = 'https://api.exchangerate-api.com/v4/latest/USD';
$jsonVeri = file_get_contents($url);
$data = json_decode($jsonVeri, true);
echo '1 USD = ' . $data['rates']['EUR'] . ' EUR';
- ✅ Bu kod, USD -> EUR döviz kurunu çeker.
3. PHP ile cURL Kullanarak API’den Veri Çekme
file_get_contents() yöntemi basit sorgular için uygundur, ancak yetkilendirme gerektiren API’lerde cURL kullanılır.cURL ile GET İsteği Yapma
PHP
$apiUrl = 'https://jsonplaceholder.typicode.com/posts/1';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
print_r($data);
- ✅ Bu kod, bir JSON API’den belirli bir gönderiyi çeker.
cURL ile POST İsteği Gönderme
PHP
$apiUrl = 'https://jsonplaceholder.typicode.com/posts';
$data = array('title' => 'Yeni Gönderi', 'body' => 'Bu bir test gönderisidir', 'userId' => 1);
$jsonData = json_encode($data);
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json'));
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
- ✅ Bu kod, API’ye yeni bir gönderi ekler.
4. PHP ile Yetkilendirme Gerektiren API Kullanımı
Bazı API’ler erişim için API Anahtarı (API Key) veya Bearer Token gerektirir.cURL ile Yetkilendirme
PHP
$apiUrl = 'https://api.example.com/protected-data';
$apiKey = 'YOUR_API_KEY';
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Authorization: Bearer $apiKey'));
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
print_r($data);
- ✅ Bu kod, API anahtarı kullanarak yetkilendirilmiş bir istekte bulunur.
5. JSON API Kullanımında Hata Yönetimi ve Güvenlik
API’lerden veri çekerken hata yönetimi yapmak ve güvenliği sağlamak çok önemlidir.Hata Yönetimi İçin cURL Kullanımı
PHP
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Hata: ' . curl_error($ch);
} else {
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode == 200) {
$data = json_decode($response, true);
print_r($data);
} else {
echo 'API isteği başarısız: HTTP Kodu $httpCode';
}
}
curl_close($ch);
- ✅ Bu kod, API’den gelen hataları kontrol eder ve HTTP yanıt kodlarını doğrular.
PHP ile JSON API kullanımı, veri alışverişini yönetmek ve dinamik uygulamalar oluşturmak için kritik bir beceridir. Bu bilgilerle PHP ve JSON API entegrasyonu konusunda daha güçlü uygulamalar geliştirebilirsiniz!
Benzer Yazılar
Yorumlar ()
Henüz yorum yok. İlk yorum yapan sen ol!